Classloader in Java
ClassLoader in Java is a class which is used to load class files in Java. Java code is compiled into class file by javac compiler and JVM executes Java program, by executing byte codes written in class file. ClassLoader is responsible for loading class files from file system, network or any other source. There are three default class loader used in Java, Bootstrap , Extension and System or Application class loader. ClassLoader main responsibilities are as below.
1) Loading
2) Linking
3) Initialization
Loading : The Class loader reads the .class file, generate the corresponding binary data and save it in method area. For each .class file, JVM stores following information in method area.
Fully qualified name of the loaded class and its immediate parent class.Whether .class file is related to Class or Interface or Enum Modifier, Variables and Method information etc. After loading .class file, JVM creates an object of type Class to represent this file in the heap memory. Please note that this object is of type Class predefined in java.lang package. This Class object can be used by the programmer for getting class level information like name of class, parent name, methods and variable information etc. To get this object reference we can use getClass() method of Object class.
Linking : Performs verification, preparation, and (optionally) resolution.
Verification : It ensures the correctness of .class file i.e. it check whether this file is properly formatted and generated by valid compiler or not. If verification fails, we get run-time exception java.lang.VerifyError.
Preparation : JVM allocates memory for class variables and initializing the memory to default values.
Resolution : It is the process of replacing symbolic references from the type with direct references. It is done by searching into method area to locate the referenced entity.
Initialization : In this phase, all static variables are assigned with their values defined in the code and static block(if any). This is executed executed from top to bottom in a class and from parent to child in class hierarchy.
In general there are three class loaders :
Bootstrap class loader : Every JVM implementation must have a bootstrap class loader, capable of loading trusted classes. It loads core java API classes present in JAVA_HOME/jre/lib directory. This path is popularly known as bootstrap path. It is implemented in native languages like C, C++.
Extension class loader : It is child of bootstrap class loader. It loads the classes present in the extensions directories JAVA_HOME/jre/lib/ext(Extension path) or any other directory specified by the java.ext.dirs system property. It is implemented in java by the sun.misc.Launcher$ExtClassLoader class.
System/Application class loader : It is child of extension class loader. It is responsible to load classes from application class path. It internally uses Environment Variable which mapped to java.class.path. It is also implemented in Java by the sun.misc.Launcher$ExtClassLoader class.
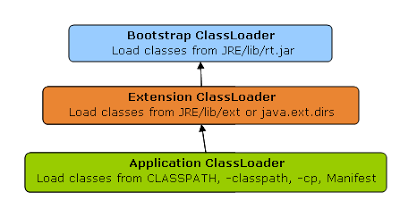
JVM follow Delegation-Hierarchy principle to load classes. System class loader delegate load request to extension class loader and extension class loader delegate request to boot-strap class loader. If class found in boot-strap path, class is loaded otherwise request again transfers to extension class loader and then to system class loader. At last if system class loader fails to load class, then we get run-time exception java.lang.ClassNotFoundException.
Custom ClassLoader :
Follow the link to learn to write your own custom classLoader.
This cover the concept of classloader in Java . If you have any question or want to add anything, then please comment below.
1) Loading
2) Linking
3) Initialization
Loading : The Class loader reads the .class file, generate the corresponding binary data and save it in method area. For each .class file, JVM stores following information in method area.
Fully qualified name of the loaded class and its immediate parent class.Whether .class file is related to Class or Interface or Enum Modifier, Variables and Method information etc. After loading .class file, JVM creates an object of type Class to represent this file in the heap memory. Please note that this object is of type Class predefined in java.lang package. This Class object can be used by the programmer for getting class level information like name of class, parent name, methods and variable information etc. To get this object reference we can use getClass() method of Object class.
Student s1 = new Student(); // Getting hold of Class object created // by JVM. Class c1 = s1.getClass(); // Printing type of object using c1. System.out.println(c1.getName());
For every loaded .class file, only one object of Class is created.
Linking : Performs verification, preparation, and (optionally) resolution.
Verification : It ensures the correctness of .class file i.e. it check whether this file is properly formatted and generated by valid compiler or not. If verification fails, we get run-time exception java.lang.VerifyError.
Preparation : JVM allocates memory for class variables and initializing the memory to default values.
Resolution : It is the process of replacing symbolic references from the type with direct references. It is done by searching into method area to locate the referenced entity.
Initialization : In this phase, all static variables are assigned with their values defined in the code and static block(if any). This is executed executed from top to bottom in a class and from parent to child in class hierarchy.
In general there are three class loaders :
Bootstrap class loader : Every JVM implementation must have a bootstrap class loader, capable of loading trusted classes. It loads core java API classes present in JAVA_HOME/jre/lib directory. This path is popularly known as bootstrap path. It is implemented in native languages like C, C++.
Extension class loader : It is child of bootstrap class loader. It loads the classes present in the extensions directories JAVA_HOME/jre/lib/ext(Extension path) or any other directory specified by the java.ext.dirs system property. It is implemented in java by the sun.misc.Launcher$ExtClassLoader class.
System/Application class loader : It is child of extension class loader. It is responsible to load classes from application class path. It internally uses Environment Variable which mapped to java.class.path. It is also implemented in Java by the sun.misc.Launcher$ExtClassLoader class.
How ClassLoader works in Java
JVM follow Delegation-Hierarchy principle to load classes. System class loader delegate load request to extension class loader and extension class loader delegate request to boot-strap class loader. If class found in boot-strap path, class is loaded otherwise request again transfers to extension class loader and then to system class loader. At last if system class loader fails to load class, then we get run-time exception java.lang.ClassNotFoundException.
Custom ClassLoader :
Follow the link to learn to write your own custom classLoader.
This cover the concept of classloader in Java . If you have any question or want to add anything, then please comment below.
Comments
Post a Comment